Using K-means Clustering to Segment Content Pages on a Website
- Aayush Maggo
- Aug 19, 2023
- 2 min read
In this post, we're diving into the world of clustering, a popular machine learning technique. Specifically, we'll explore how to use K-means clustering to segment content pages on a website. This can be incredibly useful for understanding user behavior, optimizing content delivery, and improving website design.
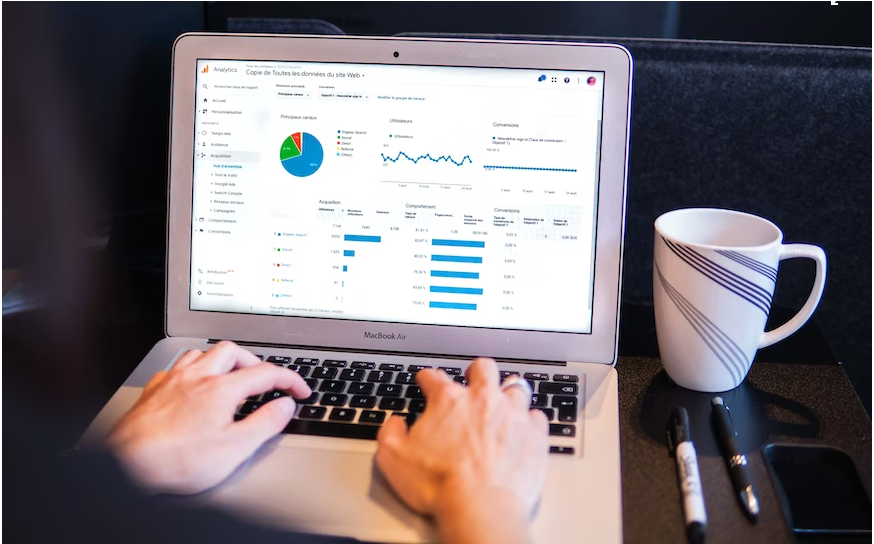
What is Clustering?
Clustering is a type of unsupervised machine learning where we group similar data points together based on certain features. Imagine you have a basket of fruits and you want to separate them based on their type. That's essentially what clustering does, but with data!
Why K-means Clustering?
K-means is one of the simplest and most popular clustering methods. It works by:
Randomly initializing 'K' cluster centers.
Assigning each data point to the nearest cluster center.
Recalculating the cluster centers based on the mean of the data points assigned to them.
Repeating steps 2 and 3 until the cluster centers no longer change significantly.
Segmenting Content Pages with K-means
Let's say you run a blog with various topics: technology, health, travel, and cooking. You want to understand which articles are similar in terms of user engagement metrics like time spent, bounce rate, and comments. K-means can help!
Step-by-Step Guide with Python Code in Colab
Data Collection First, gather data on your website's content pages. This might include metrics like:
Average time spent by users
Bounce rate
Number of comments
Number of shares
pythonCopy code
import pandas as pd
# Sample data
data = {
'Page': ['Tech1', 'Tech2', 'Health1', 'Travel1', 'Cook1'],
'Time_Spent': [5, 6, 3, 2, 4],
'Bounce_Rate': [10, 9, 15, 20, 12],
'Comments': [15, 20, 5, 3, 8],
'Shares': [20, 25, 5, 7, 10]
}
df = pd.DataFrame(data)
Data Preprocessing Normalize the data to ensure all features have equal importance.
pythonCopy code
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
scaled_data = scaler.fit_transform(df.drop('Page', axis=1))
K-means Clustering
pythonCopy code
from sklearn.cluster import KMeans
# Using the Elbow method to find the optimal number of clusters
wcss = []
for i in range(1, 5):
kmeans = KMeans(n_clusters=i, init='k-means++', random_state=42)
kmeans.fit(scaled_data)
wcss.append(kmeans.inertia_)
# Plotting the resultsimport matplotlib.pyplot as plt
plt.figure(figsize=(10,5))
plt.plot(range(1, 5), wcss, marker='o', linestyle='--')
plt.title('Elbow Method')
plt.xlabel('Number of clusters')
plt.ylabel('WCSS')
plt.show()
# Let's assume the optimal number of clusters is 3
kmeans = KMeans(n_clusters=3, init='k-means++', random_state=42)
clusters = kmeans.fit_predict(scaled_data)
df['Cluster'] = clusters
Analysis Now, you can analyze the clusters to see which content pages are grouped together and potentially why.
pythonCopy code
print(df)
Conclusion
K-means clustering offers a powerful way to segment content pages on a website, providing insights into user behavior and content performance. By understanding these segments, website owners can tailor their content strategy, improve user experience, and increase engagement.
Happy clustering! 🚀
留言