Connect GA4 Data Analytics API with Python
- Aayush Maggo
- Dec 2, 2022
- 3 min read
Google Analytics 4 (GA4) is a powerful tool for understanding user behavior and gaining valuable insights from website and app data. While the GA4 interface provides valuable reporting capabilities, many data analysts and developers prefer working with data programmatically using Python. In this article, we will explore how to connect GA4 Data Analytics API with Python to unlock the potential of advanced data analysis.
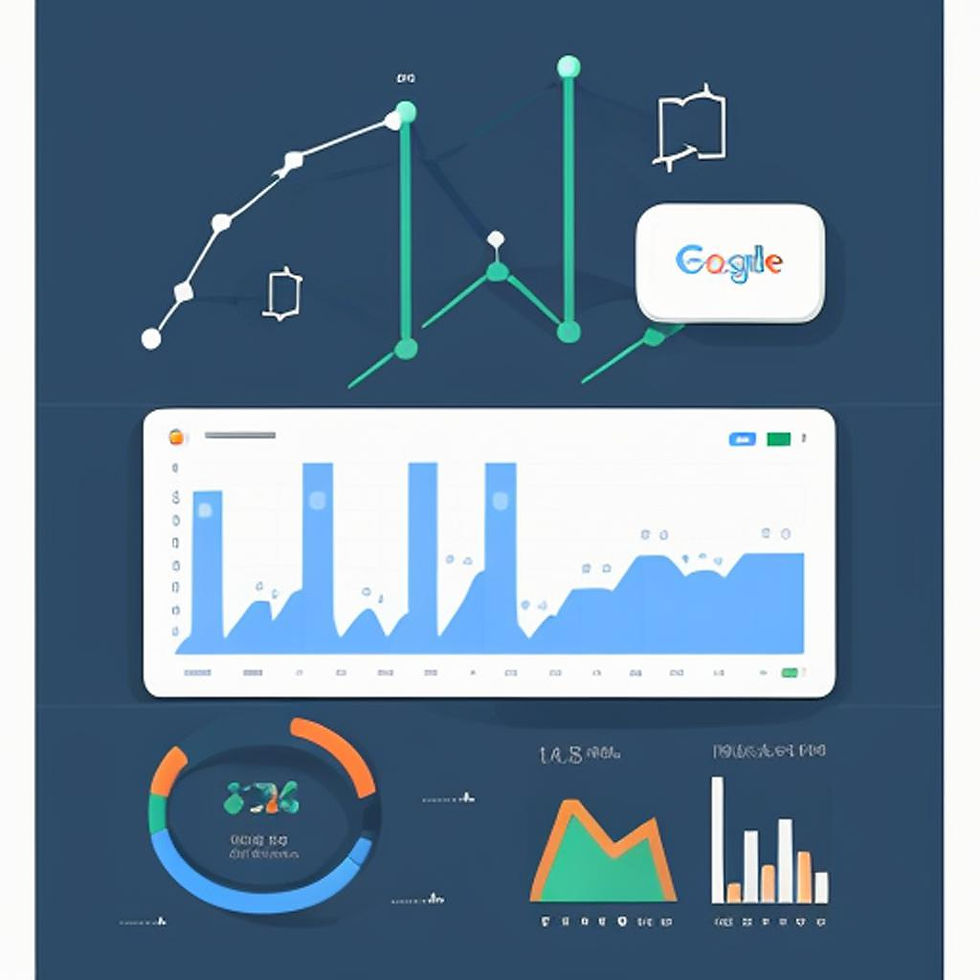
Prerequisites: Before we begin, ensure you have the following prerequisites ready:
A Google Analytics 4 property set up for your website or app.
A service account credentials JSON file downloaded from the Google Cloud Console.
Access to Google Colab or a Jupyter Notebook environment.
Step 1: Setting up Google Colab If you don't have access to Google Colab already, visit colab.research.google.com and create a new notebook. Google Colab provides an ideal environment for integrating GA4 API with Python, as it comes pre-installed with the necessary libraries and offers cloud-based computing power.
Step 2: Installing Required Libraries In Google Colab, you won't need to install any additional libraries as the environment already includes them. However, if you are using Jupyter Notebook locally, ensure you have the google-analytics-data_v1beta library installed. You can install it using the following command:
!pip install google-analytics-data_v1beta
Step 3: Authenticate the API
Replace the credentials_json_path variable in the provided code with the path to your service account credentials JSON file. This file will authenticate your requests to the GA4 API.
from google.analytics.data_v1beta import BetaAnalyticsDataClient
from google.analytics.data_v1beta.types import (
RunReportRequest,
Dimension,
Metric,
DateRange,
)
import pandas as pd
def sample_run_report(property_ids, credentials_json_path="/content/sdadadsadadas.json"):
"""
Runs a simple report on multiple Google Analytics 4 properties.
Args:
property_ids (list): A list of Google Analytics 4 property IDs.
credentials_json_path (str): The path to the credentials.json file
for your service account downloaded from the Cloud Console.
"""
# Initialize the client with service account credentials.
client = BetaAnalyticsDataClient.from_service_account_json(credentials_json_path)
# Prepare the results list to store data for each property.
results = []
for property_id in property_ids:
# Build and execute the report request for each property.
request = RunReportRequest(
property=f"properties/{property_id}",
dimensions=[Dimension(name="eventName")],
metrics=[Metric(name="eventCount")],
date_ranges=[DateRange(start_date="2023-06-28", end_date="2023-06-30")],
)
response = client.run_report(request)
# Collect data from the response and store it in the results list.
for row in response.rows:
results.append({
"Property Id": property_id,
"Event Name": row.dimension_values[0].value,
"Event Count": row.metric_values[0].value,
})
# Convert the results list to a Pandas DataFrame.
df = pd.DataFrame(results)
return df
if __name__ == "__main__":
# List of Google Analytics 4 property IDs collected from the previous code.
property_ids = property_ids_list
# Provide the path to your credentials.json file.
credentials_json_path = "/content/sdadadsadadas.json"
# Run the report for the specified properties and get the results in a DataFrame.
result_df = sample_run_report(property_ids, credentials_json_path)
Step 4:
Running the Report
In the provided code, you can see a function named sample_run_report(). This function runs a simple report on multiple Google Analytics 4 properties. Replace property_ids_list with a list of Google Analytics 4 property IDs you want to analyze.
Conclusion:
By following the steps in this guide, you have successfully connected the GA4 Data Analytics API with Python in Google Colab or Jupyter Notebook. This integration enables you to conduct advanced data analysis on your Google Analytics 4 properties, helping you understand user behavior and optimize your website or app's performance. Armed with the power of Python and the GA4 API, you can make data-driven decisions that drive growth and success for your online ventures. Happy analyzing!
Remember to save your work in Google Colab for future reference or further analysis. The data you've collected can serve as a foundation for building more complex reports and extracting deeper insights in the future.
Feel free to explore the rich functionalities of Python, Pandas, and the GA4 API to uncover even more valuable information from your Google Analytics data. The world of data analysis awaits you!
Comentarios